Hello World in Go
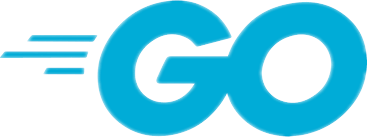
The Go programming language is an open source project to make programmers more productive. In the following blog post I assume you already have the Go environment setup. To get started with Go is just a matter of adding some code. The example below covers a basic "Hello World".
Go Installation
- Confirm Go is installed
1go version
If it is not installed check out a previous post to install Go in Linux
Development setup
With go already installed, the setup requires a couple more steps
- Create a directory for your code
- Initialise the location where the module can be found
-
Make the go home directory
1mkdir -p ~/hello && cd hello
-
Initialise the hello directory
1go mod init example.com/hello
- The directory should now look like this:
1.
2└── go.mod
Hello World
In the hello world
example we will perform three things
- Declare a package name
- Import the "fmt" package to use the fmt.Println function
- Declare a main func - which is the entry point for our go application
-
Create a hello.go program using an editor e.g.
1vi hello.go
-
Add the following code to hello.go
1package main 2import "fmt" 3func main() { 4 fmt.Println("hello, world\n") 5}
-
The directory should now look like this:
1.
2├── go.mod
3└── hello.go
Build go
The last thing to do is build our application and run it.
-
Build the hello.go application
1go build
-
The directory should now look like this:
1.
2├── go.mod
3├── hello
4└── hello.go
- Run the code hello application
1./hello
The application output should be as per below:
hello, world