Node Fake Data
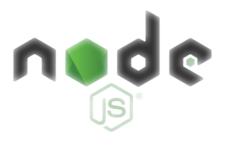
A quick guide on generating test data. If you have used the Pet Theory lab, this should be familar, but if not here is the code.
The general idea is to create a csv based on made up dataset. To do this, use the Fakerjs package and write the output as csv records.
If you have not worked with Fakerjs before, this is a nice short cut to get to grips with the Firestore API.
Requirements:
- Node.js installation
- Fakerjs package
Overview
In this blog post learn how to create fake test data in the CSV format.
Application Code
The template application will be based on Node.js. If you are unfamiliar with Node.js, this is a standard step to create the files required.
The package.json
file provides the information relating to packages used and general descriptions.
In addition it has a helpful script section, for testing that can be generalised.
- Add a package.json
1{
2 "name": "create-td",
3 "version": "1.0.0",
4 "description": "This is lab01 of the Pet Theory labs",
5 "main": "index.js",
6 "scripts": {
7 "test": "echo \"Error: no test specified\" && exit 1"
8 },
9 "keywords": [],
10 "author": "Rich Rose",
11 "license": "MIT",
12 "devDependencies": {
13 "@faker-js/faker": "^8.4.1"
14 }
15}
- Create an index.js file
The code is required to do the following things:
- Include package dependencies
- Add a createTestData function
Run app
The npm packages are required:
1npm install --save-dev @faker-js/faker
Run the application
1node index.js 10
Expected Output:
1Created file customers_10.csv containing 10 records.
Package Dependencies
A declaration for generating data.
1
2const fs = require("fs");
3const { faker } = require("@faker-js/faker");
Validate Command Line Arguments
The application expects the name of a CSV file to be added. If a filename is not present, the application will exit.
1recordCount = parseInt(process.argv[2]);
2
3if (process.argv.length != 3 || recordCount < 1 || isNaN(recordCount)) {
4 console.error("Include the number of test data records to create. Example:");
5 console.error(" node createTestData.js 100");
6 process.exit(1);
7}
8
9createTestData(recordCount);
Custom Data Schema
Use the fakerjs
package to create fake data.
The data will be written to a filename with the extension csv.
A record of the following schema is created:
Field | Type | Method |
---|---|---|
Id | Number | Number |
firstName | String | Person |
lastName | String | Person |
name | String | Person |
String | Intenet | |
phone | String | Phone |
Custom Data Generation
The createTestData
function will create the above schema.
1async function createTestData(recordCount) {
2 const fileName = `customers_${recordCount}.csv`;
3 var f = fs.createWriteStream(fileName);
4 f.write('id,name,email,phone\n')
5 for (let i=0; i<recordCount; i++) {
6 const id = faker.number.int();
7 const firstName = getFirstName(i);
8 const lastName = getLastName();
9 const phone = getPhoneNumber();
10 const name = `${firstName} ${lastName}`;
11 const email = getRandomCustomerEmail(firstName, lastName);
12 f.write(`${id},${name},${email},${phone}\n`);
13 }
14 console.log(`Created file ${fileName} containing ${recordCount} records.`);
15 f.close();
16}
Generate Email
1function getRandomCustomerEmail(fName, lName) {
2 const pName = faker.internet.domainName();
3 const email = faker.internet.email({ firstName: fName, lastName: lName, provider: pName });
4 return email.toLowerCase();
5}
Generate First Name
The following function will generate first name. The function takes an integer param, that can be used to set the gender of the name.
1function getFirstName(gender){
2 return gender%2==0 ? faker.person.firstName('female') : faker.person.firstName('male');
3}
Generate Last Name
The following function will generate a fake last name.
1function getLastName(){
2 return faker.person.lastName();
3}
Generate Phone Number
The following function will generate a fake phone number
1function getPhoneNumber(){
2 return faker.phone.number();
3}
Generated Dataset
The information below is an example dataset generated by the code.
1id,name,email,phone
21744353443708928,Hattie Shields,[email protected],994-985-4343
37439950444232704,Cornelius Douglas,[email protected],445.834.1232 x04559
43571161470337024,Ella DuBuque,[email protected],(598) 995-8925 x3962
51905977706151936,Andy Auer,[email protected],1-839-408-6820 x7671
67038984255438848,Virginia Rohan,[email protected],254.784.8123 x8950
71184946481790976,Isaac Pagac,[email protected],948-787-4669 x126
8437067337170944,Alma Windler,[email protected],(966) 478-7228
94056411071840256,Wilbert Zieme,[email protected],547.786.0668 x195
105202519139549184,Wendy Strosin,[email protected],225-558-2300
114725405374218240,Santos Koch-Bailey,[email protected],973.596.7081 x654
Complete Code
1const fs = require('fs');
2const { faker } = require('@faker-js/faker');
3
4// Function: getRandomCustomerEmail
5// Param: fName - firstName, lName - lastName
6function getRandomCustomerEmail(fName, lName) {
7 const pName = faker.internet.domainName();
8 const email = faker.internet.email({ firstName: fName, lastName: lName, provider: pName });
9
10 return email.toLowerCase();
11}
12
13// Function: getFirstName
14// Param: Gender 0 - Female, 1 - Male
15function getFirstName(gender){
16 return gender%2==0 ? faker.person.firstName('female') : faker.person.firstName('male');
17}
18
19// Function: getLastName
20// Param: N/A
21function getLastName(){
22 return faker.person.lastName();
23}
24
25// Function: getPhoneNumber
26// Param: N/A
27function getPhoneNumber(){
28 return faker.phone.number();
29}
30
31async function createTestData(recordCount) {
32 const fileName = `customers_${recordCount}.csv`;
33 var f = fs.createWriteStream(fileName);
34 f.write('id,name,email,phone\n')
35 for (let i=0; i<recordCount; i++) {
36 const id = faker.number.int();
37 const firstName = getFirstName(i);
38 const lastName = getLastName();
39 const phone = getPhoneNumber();
40 const name = `${firstName} ${lastName}`;
41 const email = getRandomCustomerEmail(firstName, lastName);
42 f.write(`${id},${name},${email},${phone}\n`);
43 }
44 console.log(`Created file ${fileName} containing ${recordCount} records.`);
45 f.close();
46}
47
48recordCount = parseInt(process.argv[2]);
49if (process.argv.length != 3 || recordCount < 1 || isNaN(recordCount)) {
50 console.error('Include the number of test data records to create. Example:');
51 console.error(' node createTestData.js 100');
52 process.exit(1);
53}
54
55createTestData(recordCount);