Node Rest API
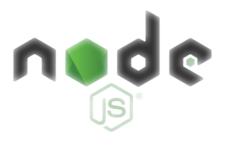
A REST API is super useful and can present a simple way to efficiently access information. This blog post is dedicated to the folks who want to create an API. It seems like a lot of folks are in this boat by the amount of comments over on sites like Reddit. Lets get started by building a GET/POST API in Node.js.
Requirements:
- Node.js installation
- Linux based environment
- Internet access
- GitHub (Optional) - code is stored there if you get stuck
Overview
In this blog post learn how to make a local REST API that response to both GET and POST requests. The following content can also be applied to a serverless environment. If you need to learn how to apply this to Google Cloud, I highly recommend reading Chapter 8 of my book Hands-On Serverless with Google Cloud.
Node.js application template
The template application will be based on Node.js. If you are unfamiliar with Node.js, this is a standard step to create the files required.
- Create a new directory for your code
1mkdir simpleapi && cd $_
- Create a package based on default settings
1npm init --y
- Add the express package to the file package.json
1npm i express
- At this point your package.json should look similar to below:
1{
2 "name": "simpleapi",
3 "version": "1.0.0",
4 "description": "",
5 "main": "index.js",
6 "scripts": {
7 "test": "echo \"Error: no test specified\" && exit 1"
8 },
9 "keywords": [],
10 "author": "",
11 "license": "ISC",
12 "dependencies": {
13 "express": "^4.17.1"
14 }
15}
- Edit the package.json file and update the script section to include a start option
1{
2 ...
3 "scripts": {
4 "start": "node index.js",
5 "test": "echo \"Error: no test specified\" && exit 1"
6 },
7 ...
8}
- Save the file
Build a REST API
At a high level a REST API is a stateless interface. It relies on HTTP operations (e.g. GET/POST) to perform tasks. In our example Node.js and Express will handle all the complicated stuff.
-
Use an editor to create a new file called
index.js
-
Add the following content to the index.js file
1const express = require('express');
2const PORT = process.env.PORT || 8080;
3const app = express();
4
5app.use(express.json());
6
7app.listen(PORT, () => {
8 console.log(`Simple REST API listening on port ${PORT}`);
9});
10
11app.get('/', async (req, res) => {
12 return res.status(200).json({'Status':'OK', 'Payload':'GET: Up and Running'});
13})
14
15app.post('/', async (req, res) => {
16 return res.status(200).json({'Status':'OK', 'Payload':'POST: Up and Running'});
17})
-
Save the file
-
The directory should now contain the following files
1.
2├── index.js
3├── node_modules
4├── package.json
5└── package-lock.json
Test the REST API
- Run the application
1npm start
-
Open a new terminal
-
Test the GET API using the following command:
1curl localhost:8080
The above command will return a response similar to below:
1{
2Status: "OK",
3Payload: "GET: Up and Running"
4}
- Test the POST api using the following command:
1curl -X POST localhost:8080
The above command will return a response similar to below:
1{
2Status: "OK",
3Payload: "POST: Up and Running"
4}
Congratulations your API is up and running!
Repository
Source Code for this blog post is available here: