Using heredoc
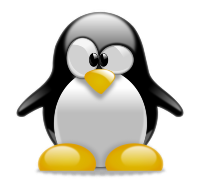
Overview
While revising for some Kubernetes exams, I realised I havent done a blog post on some useful commands.
The first blog post covers the heredoc
command.
Heredoc (Here Document) is a way to pass multiple lines of input to a command. It allows you to redirect input from a string literal within your script, instead of from a file or standard input.
The basic syntax involves using <<
followed by a delimiter. Everything
following the <<
until the delimiter is encountered again on a line by
itself is treated as input to the command. Heredocs are useful for creating
multi-line strings, embedding configuration files within scripts, and
automating interactions with command-line tools.
Use Case
Heredocs provide a convenient way to include multi-line text blocks within shell scripts. They are particularly useful for:
- Generating configuration files.
- Creating HTML or other markup files.
- Embedding SQL queries.
- Any situation where you need to work with large blocks of text in a script.
Define a heredoc
Creating a Heredoc
A heredoc starts with <<
followed by a delimiter (a word you choose).
The delimiter signals the beginning and end of the block of text.
Here's the basic structure:
1command <<DELIMITER
2 This is the content of the heredoc.
3 It can span multiple lines.
4 It can contain variables, commands and special characters.
5DELIMITER
-
This is the command that will receive the heredoc's content as input. This could be
cat
,sed
,grep
,ssh
, or any other command that reads from standard input.command
-
This operator tells the shell to redirect the subsequent text block to the standard input of
command
.DELIMITER
is a word you choose to mark the start and end of the block. It is crucial that the endingDELIMITER
appears on a line by itself, with no leading or trailing spaces or tabs. The delimiter can be any sequence of characters, but it's best to choose something unlikely to appear in the text itself. Using uppercase is a common convention.<<DELIMITER
-
This is the text that will be passed as input to the
command
. This can be any text you want, including multi-line text, shell variables, and even other commands (through command substitution, discussed later).This is the content...
HEREDOC Example
To create a simple HTML file using a shell script.
- Save the script to a file (e.g.,
create_website.sh
). - Make the script executable:
chmod +x create_website.sh
. - Run the script:
./create_website.sh
.
Example Script:
1#!/bin/bash
2
3cat << EOF > my_website.html
4<!DOCTYPE html>
5<html>
6<head>
7<title>My Simple Website</title>
8</head>
9<body>
10
11<h1>Welcome to My Website!</h1>
12<p>This is a basic HTML page created with a heredoc.</p>
13
14</body>
15</html>
16EOF
17
18echo "HTML file 'my_website.html' created."
Example with Variable Substitution:
In this example, the variables $WEBSITE_TITLE
and $HEADING_TEXT
are
expanded within the heredoc, so the resulting HTML file will contain their
values.
NOTE:
If you don't want variable substitution, you can quote the
delimiter: cat << 'EOF' ... EOF
.
Single quotes around the delimiter disable variable expansion. cat << \EOF
also works to disable variable expansion.
1#!/bin/bash
2
3WEBSITE_TITLE="My Awesome Website"
4HEADING_TEXT="Welcome, Users!"
5
6cat << EOF > my_website.html
7<!DOCTYPE html>
8<html>
9<head>
10<title>$WEBSITE_TITLE</title>
11</head>
12<body>
13
14<h1>$HEADING_TEXT</h1>
15<p>This is a dynamic HTML page created with a heredoc.</p>
16
17</body>
18</html>
19EOF
20
21echo "HTML file 'my_website.html' created."
Conclusion
Heredocs offer a clean way to pass multi-line input to commands, making scripts more readable and maintainable. They are especially useful for configuring files, sending emails, and any scenario where you need to embed a block of text directly within your script. Understanding the nuances of quoting and variable expansion is key to using them effectively.