Local JSON with Dart
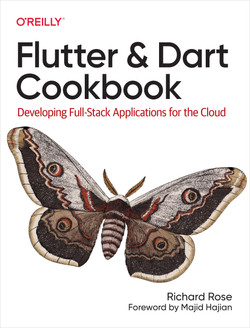
Using Dart to process JSON can seem a complicated choice at first. There are a couple of things to be aware of when processing JSON. To build our understanding I will be taking a manual approach to hand crank through a JSON file. Obviously you shouldnt do this in the real world, but this is meant to be an educational post :-) In the following blog post I assume you already have the Flutter environment setup. If you dont have an environment handy you can use dartpad.dev or similar to practice.
Local JSON
This example demonstrates loading a JSON array from a local file and displaying the data in a list.
Remember to handle potential errors, especially when dealing with file
operations and network requests in more complex scenarios. Also consider
creating data models using json_serializable
for more robust type safety in
larger projects.
-
Project Setup:
- Create a new Flutter project (if you don't already have one):
flutter create my_app
- Navigate to the project directory:
cd my_app
- Create a new Flutter project (if you don't already have one):
-
Create the JSON file:
- In the root of your project (or in an
assets
folder), create a JSON file (e.g.,data.json
). - Add some JSON data to it. For example:
- In the root of your project (or in an
1[
2 {
3 "id": 1,
4 "name": "Item 1",
5 "description": "This is the first item."
6 },
7 {
8 "id": 2,
9 "name": "Item 2",
10 "description": "This is the second item."
11 }
12]
- Update
pubspec.yaml
:- Declare the assets folder in your
pubspec.yaml
file so Flutter knows to include it in your application bundle. Add the following to yourpubspec.yaml
under theflutter:
section:
- Declare the assets folder in your
1flutter:
2 assets:
3 - data.json
4 # or if you have an assets folder
5 # - assets/data.json
* Run `flutter pub get` to fetch the updated dependencies.
- Load the JSON data:
- In your Flutter code (e.g., in
lib/main.dart
), create a function to load the JSON data from the asset file. You'll use therootBundle
class frompackage:flutter/services.dart
.
- In your Flutter code (e.g., in
1import 'dart:convert';
2import 'package:flutter/material.dart';
3import 'package:flutter/services.dart' show rootBundle;
4
5Future<String> loadJsonData() async {
6 var jsonText = await rootBundle.loadString('data.json'); // or 'assets/data.json' if in assets folder
7 return jsonText;
8}
- Parse the JSON data:
1Future<List<dynamic>> parseJsonData() async {
2 String jsonString = await loadJsonData();
3 final jsonResult = jsonDecode(jsonString);
4 return jsonResult;
5}
- Display the data in a Widget:
- Use a
FutureBuilder
to handle the asynchronous loading and parsing of the JSON data. Then display the content.
- Use a
1class MyHomePage extends StatelessWidget {
2 const MyHomePage({Key? key}) : super(key: key);
3
4 @override
5 Widget build(BuildContext context) {
6 return Scaffold(
7 appBar: AppBar(
8 title: const Text('Local JSON Example'),
9 ),
10 body: FutureBuilder<List<dynamic>>(
11 future: parseJsonData(),
12 builder: (BuildContext context, AsyncSnapshot<List<dynamic>> snapshot) {
13 if (snapshot.hasData) {
14 return ListView.builder(
15 itemCount: snapshot.data!.length,
16 itemBuilder: (BuildContext context, int index) {
17 return ListTile(
18 title: Text(snapshot.data![index]['name']),
19 subtitle: Text(snapshot.data![index]['description']),
20 );
21 },
22 );
23 } else if (snapshot.hasError) {
24 return Center(child: Text('Error: ${snapshot.error}'));
25 } else {
26 return const Center(child: CircularProgressIndicator());
27 }
28 },
29 ),
30 );
31 }
32}
- Complete
main.dart
:- Wrap it all up in the standard
main.dart
file:
- Wrap it all up in the standard
1import 'dart:convert';
2import 'package:flutter/material.dart';
3import 'package:flutter/services.dart' show rootBundle;
4
5Future<String> loadJsonData() async {
6 var jsonText = await rootBundle.loadString('data.json');
7 return jsonText;
8}
9
10Future<List<dynamic>> parseJsonData() async {
11 String jsonString = await loadJsonData();
12 final jsonResult = jsonDecode(jsonString);
13 return jsonResult;
14}
15
16void main() {
17 runApp(const MyApp());
18}
19
20class MyApp extends StatelessWidget {
21 const MyApp({Key? key}) : super(key: key);
22
23 @override
24 Widget build(BuildContext context) {
25 return MaterialApp(
26 title: 'Flutter Local JSON Demo',
27 theme: ThemeData(
28 primarySwatch: Colors.blue,
29 ),
30 home: const MyHomePage(),
31 );
32 }
33}
34
35class MyHomePage extends StatelessWidget {
36 const MyHomePage({Key? key}) : super(key: key);
37
38 @override
39 Widget build(BuildContext context) {
40 return Scaffold(
41 appBar: AppBar(
42 title: const Text('Local JSON Example'),
43 ),
44 body: FutureBuilder<List<dynamic>>(
45 future: parseJsonData(),
46 builder: (BuildContext context, AsyncSnapshot<List<dynamic>> snapshot) {
47 if (snapshot.hasData) {
48 return ListView.builder(
49 itemCount: snapshot.data!.length,
50 itemBuilder: (BuildContext context, int index) {
51 return ListTile(
52 title: Text(snapshot.data![index]['name']),
53 subtitle: Text(snapshot.data![index]['description']),
54 );
55 },
56 );
57 } else if (snapshot.hasError) {
58 return Center(child: Text('Error: ${snapshot.error}'));
59 } else {
60 return const Center(child: CircularProgressIndicator());
61 }
62 },
63 ),
64 );
65 }
66}
- Run the application:
flutter run
Conclusion
Handling JSON in Flutter/Dart is a common and crucial task when building
applications that interact with APIs or local data. By mastering techniques
like serialization/deserialization with dart:convert
, code generation
using json_serializable
and build_runner
, and understanding different
strategies for handling complex JSON structures, you can efficiently manage
data and build robust, maintainable Flutter applications. Remember to choose
the method that best suits the complexity of your JSON data and the
performance requirements of your application. Consider error handling and
data validation to ensure the reliability and integrity of your data. With
practice, you'll become comfortable handling various JSON scenarios in your
Flutter development.